How to convert an uploaded data table to a data frame in R?
Let’s say I uploaded a dataset to R.
#install.packages("readr")
library(readr)
github="https://raw.githubusercontent.com/agronomy4future/raw_data_practice/main/soybean_yield.csv"
dataA=data.frame(read_csv(url(github), show_col_types=FALSE))
dataA
season crop plot treatment grain_yield
1 2023 Soybean 1 tr1 32.2
2 2023 Soybean 1 tr2 5.0
3 2023 Soybean 1 tr3 4.3
4 2023 Soybean 1 tr3 1.0
5 2023 Soybean 1 tr5 8.7
6 2023 Soybean 2 tr1 25.6
7 2023 Soybean 2 tr2 35.7
8 2023 Soybean 2 tr3 29.2
9 2023 Soybean 2 tr3 39.9
10 2023 Soybean 2 tr5 20.7
11 2023 Soybean 3 tr1 15.5
12 2023 Soybean 3 tr2 23.2
13 2023 Soybean 3 tr3 44.6
14 2023 Soybean 3 tr3 45.0
15 2023 Soybean 3 tr5 32.4
16 2023 Soybean 4 tr1 20.5
17 2023 Soybean 4 tr2 35.2
18 2023 Soybean 4 tr3 37.3
19 2023 Soybean 4 tr3 9.6
20 2023 Soybean 4 tr5 16.8
21 2023 Soybean 5 control 103.2
22 2023 Soybean 6 control 60.8
23 2023 Soybean 7 control 33.0
24 2023 Soybean 8 control 66.2
Now, I want to save this data as code so that I can store it in my web note. This is because it would be difficult to find the original dataset after a long time. Therefore, I want to save it as text code in a list on my web note.
1) using dput()
First, we can use dput()
function.
dput(dataA)
structure(list(season = c(2023, 2023, 2023, 2023, 2023, 2023,
2023, 2023, 2023, 2023, 2023, 2023, 2023, 2023, 2023, 2023, 2023,
2023, 2023, 2023, 2023, 2023, 2023, 2023), crop = c("Soybean",
"Soybean", "Soybean", "Soybean", "Soybean", "Soybean", "Soybean",
"Soybean", "Soybean", "Soybean", "Soybean", "Soybean", "Soybean",
"Soybean", "Soybean", "Soybean", "Soybean", "Soybean", "Soybean",
"Soybean", "Soybean", "Soybean", "Soybean", "Soybean"), plot = c(1,
1, 1, 1, 1, 2, 2, 2, 2, 2, 3, 3, 3, 3, 3, 4, 4, 4, 4, 4, 5, 6,
7, 8), treatment = c("tr1", "tr2", "tr3", "tr3", "tr5", "tr1",
"tr2", "tr3", "tr3", "tr5", "tr1", "tr2", "tr3", "tr3", "tr5",
"tr1", "tr2", "tr3", "tr3", "tr5", "control", "control", "control",
"control"), grain_yield = c(32.2, 5, 4.3, 1, 8.7, 25.6, 35.7,
29.2, 39.9, 20.7, 15.5, 23.2, 44.6, 45, 32.4, 20.5, 35.2, 37.3,
9.6, 16.8, 103.2, 60.8, 33, 66.2)), class = "data.frame", row.names = c(NA,
-24L))
2) using datapasta()
Second, we can use datapasta()
function
#install.packages("datapasta")
library(datapasta)
datapasta::df_paste(dataA)
data.frame(
stringsAsFactors=FALSE,
season=c(2023,2023,2023,2023,2023,
2023,2023,2023,2023,2023,2023,2023,2023,2023,2023,
2023,2023,2023,2023,2023,2023,2023,2023,2023),
crop=c("Soybean","Soybean","Soybean",
"Soybean","Soybean","Soybean","Soybean","Soybean",
"Soybean","Soybean","Soybean","Soybean","Soybean",
"Soybean","Soybean","Soybean","Soybean","Soybean",
"Soybean","Soybean","Soybean","Soybean","Soybean",
"Soybean"),
plot=c(1,1,1,1,1,2,2,2,2,2,3,3,3,3,3,4,4,4,4,4,5,6,7,8),
treatment=c("tr1","tr2","tr3","tr3","tr5","tr1","tr2",
"tr3","tr3","tr5","tr1","tr2","tr3","tr3",
"tr5","tr1","tr2","tr3","tr3","tr5",
"control","control","control","control"),
grain_yield=c(32.2,5,4.3,1,8.7,25.6,35.7,29.2,39.9,20.7,
15.5,23.2,44.6,45,32.4,20.5,35.2,37.3,9.6,
16.8,103.2,60.8,33,66.2)
)
3) using constructive()
This is a great package from https://cynkra.github.io/constructive. The best thing about this package is that the code is simplified by using rep()
.
#install.packages("remotes")
#install.packages("constructive")
#remotes::install_github("cynkra/constructive")
library(remote)
constructive::construct(dataA)
data.frame(
season=rep(2023, 24L),
crop=rep("Soybean", 24L),
plot=rep(seq(1, 8, by=1), rep(c(5L, 1L), each=4L)),
treatment=c("tr1", "tr2", "tr3", "tr3", "tr5", "tr1", "tr2",
"tr3", "tr3", "tr5", "tr1", "tr2", "tr3", "tr3",
"tr5", "tr1", "tr2", "tr3", "tr3", "tr5", "control",
"control", "control", "control"),
grain_yield=c(32.2, 5, 4.3, 1, 8.7, 25.6, 35.7, 29.2, 39.9, 20.7,
15.5, 23.2, 44.6, 45, 32.4, 20.5, 35.2, 37.3, 9.6,
16.8, 103.2, 60.8, 33, 66.2)
)
Reference
https://stackoverflow.com/questions/77834006/how-to-convert-uploaded-data-table-to-data-frame-code-in-r
Code summary
https://github.com/agronomy4future/r_code/blob/main/How_to_convert_uploaded_data_table_to_data_frame()_code_in_R%3F.ipynb
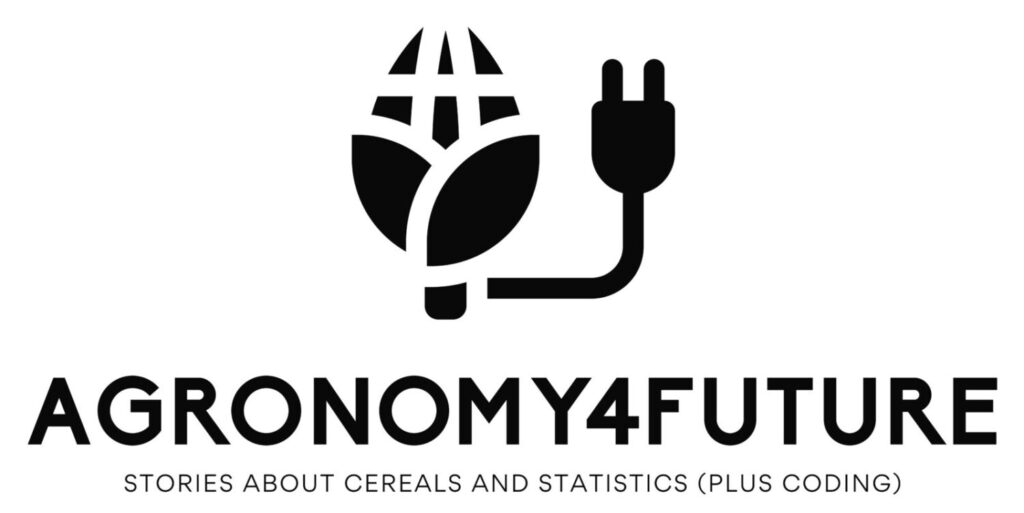
© 2022 – 2023 https://agronomy4future.com